Serve Fine-tuned LLMs with Ollama
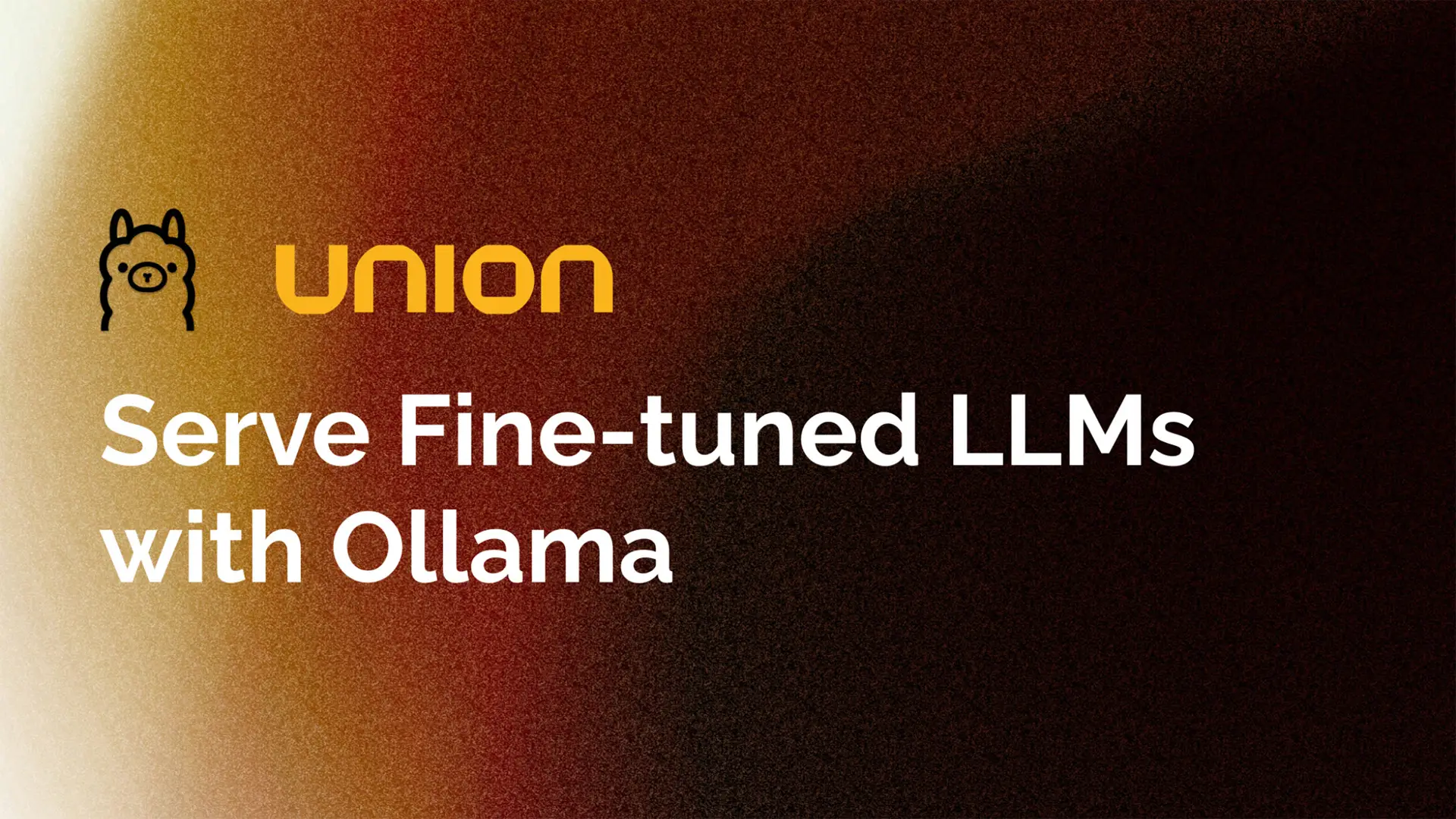
Ollama is a platform designed to simplify running open-source large language models (LLMs) locally. Deploying LLMs is often challenging due to varying model configurations and the lack of a standardized serving method. Ollama takes the headache out of this process by packaging everything you need to run an LLM—from model weights to system prompts—into a single, easy-to-use modelfile. The best part? Serving these models is incredibly straightforward. You can spin up a server, pull or create a custom model, and instantly start generating predictions.
With the Ollama plugin, serving fine-tuned models becomes even more efficient. Whether you’re fine-tuning a model for further evaluation or deploying an LLM for downstream tasks, the plugin makes it easy to integrate Ollama into Flyte tasks without the usual complexities of orchestrating serving infrastructure. Just instantiate the plugin, and you’ll have direct access to your model right within your Flyte task. It’s really that simple!
Simplify and accelerate AI model fine-tuning and serving with full control over infrastructure, costs, and data security
To truly appreciate what the Ollama plugin offers, it's helpful to understand what challenges exist without it.
While Ollama is a powerful tool on its own—and, in my experience, incredibly easy to set up—there are specific scenarios where the plugin really shines:
- Serving models on general-purpose hardware: After fine-tuning your model on a GPU, you might need to see how it performs on other types of hardware, whether accelerated or general-purpose. You should be able to switch between these hardware options with ease.
- Running batch inference within your AI pipeline: Integrate batch inference into your AI pipelines.
In the first scenario, fine-tuning on a GPU is often essential, but it can be cumbersome to handle fine-tuning and model serving in two separate environments. The Ollama plugin simplifies this by allowing you to preprocess data, fine-tune your model, and generate predictions all within a single, cohesive pipeline. This means you can serve your model right after fine-tuning, without the usual infrastructure hassles. Union abstracts away the complexity of fine-tuning, while the plugin allows you to serve models locally within a Flyte task, minimizing network overhead. Even better, you can easily configure different hardware for model serving, giving you full flexibility and control.
The second scenario highlights batch inference, which is becoming a staple in AI pipelines due to its cost efficiency compared to real-time inference. Real-time processing isn’t always necessary, and batch inference offers a practical alternative. With Union’s orchestration capabilities, you can easily integrate batch inference using the Ollama plugin, serving both pre-trained and custom LLMs. You can also build Retrieval Augmented Generation (RAG) batch inference pipelines with ease using this plugin.
This plugin enables you to self-host and serve optimized AI models on your own infrastructure powered by Union, ensuring full control over costs and data security. By eliminating dependence on third-party APIs for AI model access, you gain not only enhanced control but also potentially lower expenses compared to traditional API services.
Union also gives you the flexibility to serve multiple Ollama models at the same time, each with its own configuration on different instances. This setup allows you to:
- Boost throughput with parallel processing: Handle multiple Ollama sidecar container tasks simultaneously for better performance.
- Build specialized applications with ease: Create and manage a variety of tasks within a single pipeline, tailored to your specific needs.
Fine-tune and serve Phi3 model
The plugin is best understood through a practical example. Let’s walk through the process of creating a PubMed dataset, fine-tuning a Phi3 model, and then serving it. The source code of this workflow can be found here.
The dataset creation can be handled in a single Flyte task.
Next, we’ll fine-tune the model on a GPU, allocating the necessary resources to ensure optimal performance.
Once fine-tuning is complete, the task returns two directories: one containing the LoRA adapter and the other containing the fine-tuned model.
To serve the model with Ollama, the LoRA adapter must be converted to the GGUF format. Alternatively, you can opt to convert it into the Safetensors format, depending on your deployment needs.
We’ll then instantiate the `Ollama` class, derived from `flytekitplugins.inference`. Make sure to install the plugin first using the command: `pip install flytekitplugins-inference`.
In the code, we define a `modelfile` to reference the fine-tuned model along with a custom system prompt. Flyte will dynamically materialize `{inputs.gguf}` during runtime, and the `gguf` input corresponds to the task. From the instantiated object, you can access the base URL and use it to hit the endpoint for generating predictions.
All these tasks can be encapsulated within a Flyte workflow, as demonstrated below:
Union makes managing Ollama workflows a breeze by handling the entire lifecycle. Here’s how it benefits you:
- Single, Cohesive Pipeline: Bring everything together in one Flyte workflow. From data processing to model serving, keep everything in one place without having to deal with disconnected pieces.
- Per-Task Resource Allocation: Customize how much CPU, GPU, and memory each task gets. You can allocate more resources for heavy lifting like fine-tuning and scale back for lighter tasks like data processing and model serving.
- Caching: Save time and avoid redundant work by caching tasks with the same inputs. This means you won’t have to repeat tasks unnecessarily, making your workflow more efficient.
Some sections of the code in this post were contributed by Niels Bantilan.
Start serving your models today
Ready to dive in? With the Ollama plugin, you can start serving your fine-tuned models right away. It’s easy to generate batch predictions by serving models locally within Flyte tasks. Plus, you can plug Ollama into various stages of your AI workflow, from data pre-processing to model inference and even post-processing and analysis.
Thinking about building production-grade AI pipelines? Feel free to reach out to the Union team—we’re here to help you make it happen.